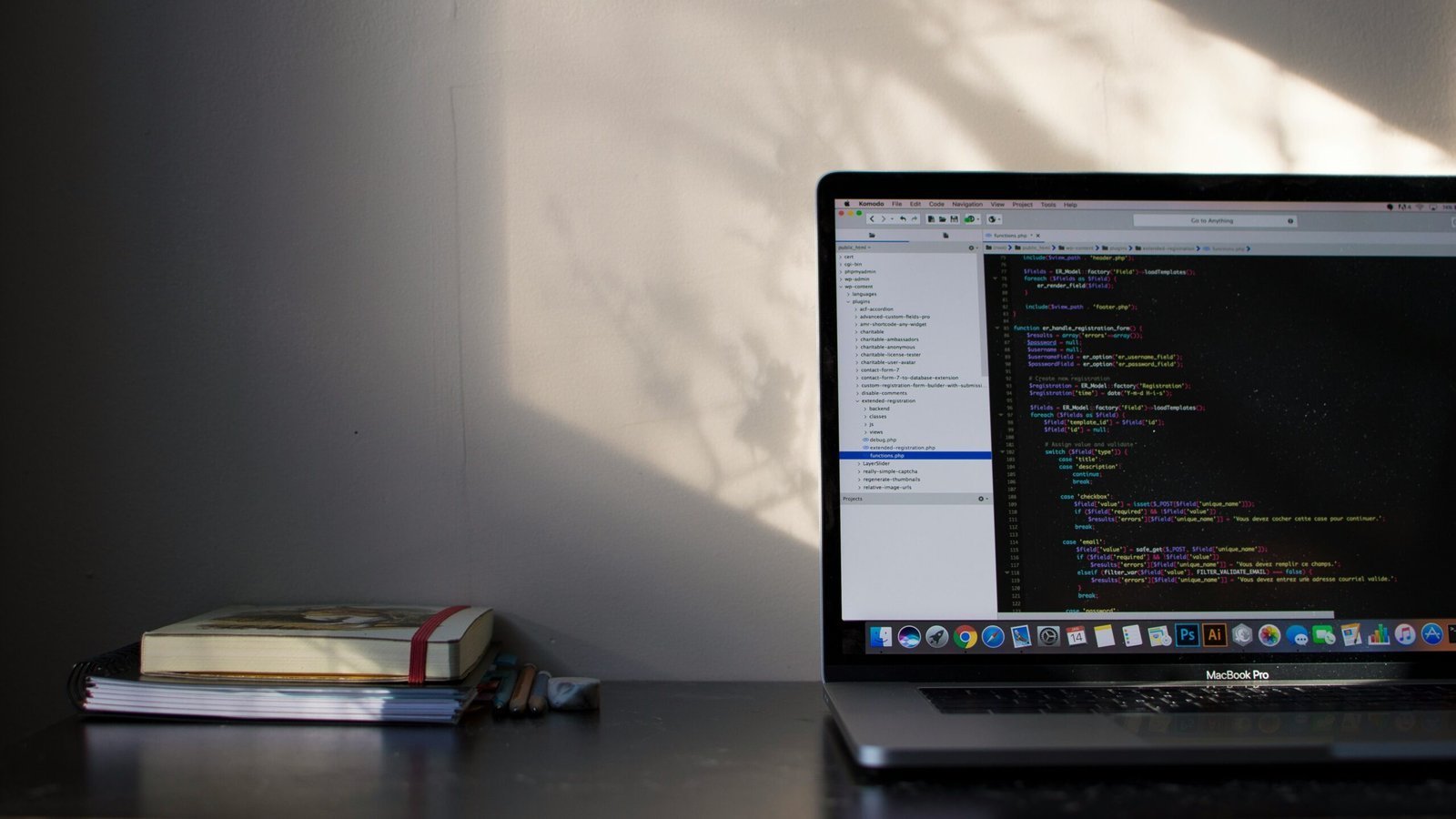
Introduction to C/C++
C/C++(C and C++) are prominent programming languages that have played a substantial role in the evolution of software development. C, developed in the early 1970s by Dennis Ritchie at Bell Labs, has often been described as the “mother” of modern programming languages. Its clarity and efficiency made it the choice for systems programming. On the other hand, C++ was introduced in the early 1980s by Bjarne Stroustrup as an enhancement to C, incorporating object-oriented features to facilitate code reuse and modularity. These enhancements have enabled developers to implement complex systems more efficiently.
Both languages are characterized by their performance and control over system resources, which is critical for building powerful and efficient programs. In C, developers deal with low-level operations, granting them direct access to memory management. C++, while retaining the same features as C, adds abstraction and encapsulation, making it suitable for larger software projects where the benefits of object-oriented programming can be fully realized.
It is essential to understand the key differences between C and C++. While C is procedural and focuses on function calls, C++ supports both procedural and object-oriented paradigms, allowing for more flexible design in programming. This distinction makes C++ preferable for applications requiring extensive codebases, such as game development and large-scale software systems. Conversely, C is widely used for embedded systems and operating system kernels, where low-level hardware interaction is paramount.
Due to their robust nature, C and C++ find applications in several domains, including systems programming, game development, and high-performance applications. These languages are foundational skills for aspiring software developers, as mastering C/C++ enables them to build powerful and efficient programs across a broad spectrum of industries.
Setting Up Your Development Environment
Creating a robust development environment is a fundamental step in mastering C/C++. A well-configured setup enhances productivity and fosters an efficient programming experience, which is essential for building powerful and effective programs. The first component to consider is the Integrated Development Environment (IDE). Popular choices include Visual Studio, Code::Blocks, and Eclipse. Each of these options offers unique features and tools that cater to different programming styles and preferences.
Visual Studio, for instance, is widely recognized for its comprehensive features and user-friendly interface. It is particularly advantageous for developers working on Windows, as it provides extensive support for C/C++ development, along with built-in debugging tools and a powerful compiler. Alternatively, Code::Blocks is an open-source IDE that supports multiple compilers and is viewed favorably by many due to its simplicity and flexibility. Eclipse, another powerful IDE, is popular for its extensibility through plugins and is suitable for those who prefer a more customizable environment.
In addition to IDEs, having the right compiler is crucial in mastering C/C++. GCC (GNU Compiler Collection) is a widely used open-source compiler that supports various platforms, making it an excellent choice for cross-platform development. Clang is another modern compiler renowned for its performance and error-checking capabilities. Integrating these tools effectively can significantly streamline the process of coding and debugging.
Moreover, incorporating debugging tools and version control systems is vital for managing project complexities as you develop powerful and efficient programs. Tools like GDB for debugging and Git for version control help maintain code quality and facilitate collaborative efforts. Overall, investing time in setting up your development environment is essential in laying a strong foundation for mastering C/C++.
Fundamentals of C/C++ Programming
Understanding the fundamentals of C and C++ programming is essential for anyone aiming at mastering C/C++. These languages are foundational in the realm of software development, and their core concepts allow programmers to build powerful and efficient programs. At the heart of C/C++ are variables and data types, which serve as the building blocks for data manipulation. Variables store data that can be referenced and modified throughout the program. Data types, such as integers, floating-point numbers, and characters, specify the kind of data that can be stored, influencing how much memory is allocated and how operations are performed.
Control structures are another critical aspect of programming in C/C++. They dictate the flow of execution of a program. Utilizing if-else statements, loops, and switch cases effectively enables programmers to create dynamic and responsive applications. Differences between the C and C++ syntax can also lead to improved program performance and organization—C++ supports additional features such as classes and objects that aid in structuring programs more efficiently.
Functions are a vital component in both languages, allowing developers to encapsulate code that performs specific tasks. This modularity not only promotes code reuse but also enhances global program readability, a key to mastering C/C++. Furthermore, an understanding of memory management is paramount. C/C++ programming involves the distinction between stack and heap memory allocations. The stack is used for static memory allocation, while the heap is utilized for dynamic memory allocation—both concepts are crucial when it comes to optimizing program performance and resource usage. By grasping these fundamental elements, aspiring programmers set a strong foundation for tackling more complex topics in C/C++ programming, ultimately leading to the ability to build powerful and efficient programs.
Object-Oriented Programming in C++
Object-oriented programming (OOP) is a cornerstone of mastering C/C++, enabling developers to create complex applications that are both powerful and efficient. In C++, OOP principles such as classes, objects, inheritance, polymorphism, and encapsulation allow developers to build modular and reusable code. This section will explore these fundamental concepts in detail.
A class in C++ serves as a blueprint for creating objects. It encapsulates data and functions that manipulate that data. By defining a class, developers can represent real-world entities more effectively, promoting an organized coding structure. For example, consider a class named ‘Car’ that encapsulates attributes like ‘speed’ and ‘fuel consumption’ and methods to operate the vehicle. This encapsulation of functionality not only clarifies the program structure but also enhances code maintenance.
Inheritance allows developers to create new classes based on existing ones, establishing a hierarchical relationship between classes. This promotes code reuse, as properties and behaviors of the parent class can be inherited by the child class. For instance, if a ‘Vehicle’ class exists, a ‘Truck’ class can derive from it and gain access to the shared functionalities, thus avoiding redundancy and ensuring program efficiency.
Another vital feature of C++ is polymorphism, which enables methods to do different things based on the object invoking them. This is useful in scenarios where a function operates on different classes but requires flexibility in implementation. Polymorphism enhances the extensibility of the code, making it adaptable to new requirements.
Encapsulation further safeguards data integrity by restricting direct access to an object’s state. Instead, interaction occurs through public methods, ensuring that objects maintain a valid state throughout their lifecycle. By applying these object-oriented principles, programmers can effectively enhance not only the structural integrity of the code but also its overall maintainability and efficiency in programming.
Memory Management and Pointers
Memory management is a fundamental concept in the realm of C/C++. As programming languages that offer developers low-level access to system resources, both C and C++ require a comprehensive understanding of how memory operates, particularly through the use of pointers. Pointers serve as a means to refer to memory locations directly, enabling programmers to manipulate the contents of these locations effectively. This direct access facilitates dynamic memory allocation, which is essential for building powerful and efficient programs.
Dynamic allocation occurs when a program requests memory from the operating system during runtime. Functions such as malloc
, calloc
, and free
in C, or the new
and delete
operators in C++, allow for this capability. However, manual memory management also carries risks. Improperly managed memory can lead to leaks and overhead that degrade performance, ultimately affecting the program’s efficiency. A memory leak happens when allocated memory is not released back to the system after use, leading to gradual memory consumption without recuperation.
Another common issue is the occurrence of dangling pointers, which refer to memory locations that have already been deallocated. Accessing such pointers can result in undefined behavior, which poses serious risks for software stability and integrity. To avoid these pitfalls, developers must implement careful coding practices, ensuring that every allocated memory block is appropriately freed once it is no longer needed. Using smart pointers in C++ can help alleviate some of the manual management burdens, as they automatically manage memory lifetimes.
Furthermore, debugging tools, such as Valgrind, can significantly aid in identifying and resolving memory issues. By thoroughly analyzing memory usage, Valgrind allows developers to detect leaks and improper memory management practices, fostering the creation of robust applications. Therefore, mastering C/C++ demands a firm grasp of memory management techniques and an awareness of the potential entanglements that come with pointer usage.
Advanced C/C++ Features
Mastering C/C++ involves exploring its advanced features that enhance the language’s capability to build powerful and efficient programs. Two prominent features that significantly contribute to programming efficiency are templates and the Standard Template Library (STL). Templates allow developers to create functions and classes that operate with any data type, thereby promoting code reusability and flexibility. For example, a single function template can sort various data types without writing separate functions for each type. This not only streamlines the development process but also reduces redundancy, resulting in more maintainable code.
The Standard Template Library (STL) complements templates by providing a robust collection of data structures and algorithms that are well-optimized for performance. STL includes a variety of containers, such as vectors, lists, and maps, each designed to address specific performance and memory usage needs. By utilizing STL, programmers can implement complex data handling tasks more efficiently, allowing them to focus on developing features rather than reinventing data structures. The availability of generic algorithms, such as sort and find, further empowers developers to construct effective solutions with minimal effort.
Another critical aspect of mastering C/C++ features is exception handling. This mechanism enables developers to manage errors gracefully, ensuring that programs can handle unexpected situations without crashing. By leveraging try-catch blocks, programmers can define specific actions to take when an exception occurs, thus enhancing code robustness. Implementing best practices in exception handling, such as avoiding using exceptions for flow control and ensuring exceptions do not leak resources, contribute to writing efficient and resilient code.
Lastly, the use of smart pointers introduces an advanced method for managing dynamic memory. Unlike traditional pointers, smart pointers automatically handle memory allocation and deallocation, significantly reducing memory leaks and dangling pointers. By incorporating these advanced C/C++ features, programmers can attain a higher standard of code quality, enabling them to build powerful and efficient programs with greater proficiency.
Building and Compiling C/C++ Programs
Building and compiling C/C++ programs is a critical aspect of developing efficient and powerful applications. The process typically involves translating source code into machine code that a computer can execute. To facilitate this, developers often utilize various tools and techniques, resulting in smoother workflows and enhanced productivity.
A foundational element in this process is the Makefile. A Makefile is a special file that dictates how to compile and link the program. By specifying dependencies between files, developers can automate the build process. This automation ensures that only modified files are recompiled, significantly reducing compilation time. Understanding the structure and syntax of Makefiles is essential for mastering C/C++, as it allows for the management of complex projects with multiple source files efficiently.
In addition to Makefiles, automated build systems like CMake and Meson have gained popularity. These systems offer advanced features, such as cross-platform compatibility and the ability to manage dependencies more effectively. Utilizing an automated build system streamlines the compilation process and reduces the potential for human error during manual builds. As development teams grow, employing these systems becomes vital for maintaining code integrity and ensuring that builds are consistently reproducible.
Continuous Integration (CI) practices further enhance the development workflow. By incorporating CI, developers can automatically build and test their C/C++ programs every time a change is made to the codebase. This practice not only accelerates the feedback loop but also aids in maintaining code quality, as bugs can be identified and addressed promptly. Effective CI processes help ensure that programs are reliably built and meet quality standards before deployment.
Optimizing the compilation process is also key to building powerful and efficient programs. Techniques such as precompiled headers, parallel compilation, and utilizing more efficient compiler flags can lead to faster build times. Coupling these optimizations with proper testing and quality assurance practices ensures that the final output is robust and reliable.
Debugging and Testing Techniques
Debugging is a vital component of software development, especially in languages such as C and C++. Given their complexity and low-level operations, mastering C/C++ requires familiarity with effective debugging tools and techniques. One of the most widely used debugging tools is the GNU Debugger, commonly known as gdb. This powerful command-line utility allows developers to inspect what is happening inside a program while it executes or during its crash. With gdb, programmers can set breakpoints, examine variables, and control the execution flow, which is essential for diagnosing and resolving underlying issues in their code.
Moreover, many Integrated Development Environments (IDEs) come equipped with built-in debuggers that simplify the debugging process. IDEs such as Code::Blocks, Visual Studio, and Eclipse provide graphical interfaces that allow developers to set breakpoints, watch variables, and step through code more intuitively. This user-friendly design can significantly enhance productivity, making the debugging phase less daunting and more efficient.
In addition to utilizing debugging tools, following best practices can help developers systematically track down bugs. Techniques such as logging, code reviews, and pair programming are effective ways to identify potential issues early in the development cycle. Logging important interactions and variable states can provide insights into unexpected behavior during execution. Furthermore, adopting a rigorous code review practice promotes collective code quality, as peers can catch issues that may not be evident to the original developer.
Testing is also crucial to ensuring the reliability of software built in C/C++. Implementing a unit testing framework, such as Google Test, allows developers to write tests for their functions, ensuring that programs work as intended. By integrating testing into the development process, developers can validate their code continually and maintain confidence in their programs. This proactive approach to debugging and testing is essential for mastering C/C++ and ultimately achieving the creation of powerful and efficient programs.
Best Practices for Writing Efficient Programs
Efficient coding in C/C++ is fundamental to mastering these languages and building powerful and efficient programs. To achieve this, developers should adopt several best practices that focus on both performance and maintainability. One crucial aspect is code organization. Clear structuring of code enhances readability and makes it easier to navigate, especially in larger projects. By using consistent naming conventions and breaking down code into smaller, manageable functions or modules, programmers can significantly improve the maintainability of their applications.
Optimization techniques play a critical role in creating efficient programs. It is essential to understand the various optimizations available in C/C++ and apply them judiciously. This includes selecting appropriate algorithms and data structures, as well as leveraging compiler optimizations. However, it is important to strike a balance between optimization and code clarity; over-optimization can lead to complicated code that is difficult to understand and maintain. Therefore, focusing on writing clean and simple code should be a priority while optimizing for performance.
Another effective practice is conducting regular code reviews. Engaging peers in reviewing code not only helps identify potential inefficiencies or bugs but also fosters knowledge sharing among team members. Such collaboration can lead to improved coding techniques and solidifies the understanding of best practices, which is invaluable for those mastering C/C++ and striving to build powerful and efficient programs.
Moreover, writing self-documenting code is an often-overlooked strategy that can enhance the quality of the software. This involves using meaningful variable and function names that convey the purpose of the code without the need for extensive comments. This practice aids in maintaining clarity and understanding as the code evolves over time.
Implementing these best practices can greatly enhance programming skills and result in the development of high-quality software that is both powerful and efficient.
- Name: Mohit Anand
- Phone Number: +91-9835131568(Sumit Singh)
- Email ID: teamemancipation@gmail.com
- Our Platforms:
- Digilearn Cloud
- EEPL Test
- Live Emancipation
- Follow Us on Social Media:
- Instagram – EEPL Classroom
- Facebook – EEPL Classroom
- Go back to google
Internal links
- Building Your First Python Project: A Step-by-Step Guide
- Python vs. Other Languages: Why It’s the Best Choice for Beginners
- Artificial Intelligence in the Future: Trends, Challenges & Opportunities
- Transforming Healthcare with AI: Faster Diagnosis and Better Treatment
- The Future of AI in Business: Smarter, Faster, Better
- The Truth About AI Ethics: Challenges, Risks, and Real Solutions
- The Future of AI and Data Science: Smarter, Faster, Better
- ML vs. DL: Exploring Their Unique Roles in AI